Recently I had a need for a simple progress bar written in VBScript to let users know what was going on in a multi-step application. To my surprise, VBScript has nothing built in for a progress bar. After much searching, I was able to find this thread that linked to yet another thread with a simple progress bar done in HTML using InternetExplorer.Application. I have taken this code and modified it to show an actual progess bar, with percentages, that can be updated as desired. I’ve wrapped it up in a nice easy-to-use class for reusability. Enjoy!
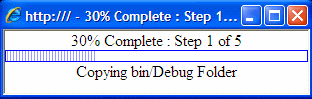
Simple VBScript Progress Bar
VBScript Progress Bar Class:
Class ProgressBar Private m_PercentComplete Private m_CurrentStep Private m_ProgressBar Private m_Title Private m_Text Private m_StatusBarText 'Initialize defaults Private Sub ProgessBar_Initialize m_PercentComplete = 0 m_CurrentStep = 0 m_Title = "Progress" m_Text = "" End Sub Public Function SetTitle(pTitle) m_Title = pTitle End Function Public Function SetText(pText) m_Text = pText End Function Public Function Update(percentComplete) m_PercentComplete = percentComplete UpdateProgressBar() End Function Public Function Show() Set m_ProgressBar = CreateObject("InternetExplorer.Application") 'in code, the colon acts as a line feed m_ProgressBar.navigate2 "about:blank" : m_ProgressBar.width = 315 : m_ProgressBar.height = 40 : m_ProgressBar.toolbar = false : m_ProgressBar.menubar = false : m_ProgressBar.statusbar = false : m_ProgressBar.visible = True m_ProgressBar.document.write "<body Scroll=no style='margin:0px;padding:0px;'><div style='text-align:center;'><span name='pc' id='pc'>0</span></div>" m_ProgressBar.document.write "<div id='statusbar' name='statusbar' style='border:1px solid blue;line-height:10px;height:10px;color:blue;'></div>" m_ProgressBar.document.write "<div style='text-align:center'><span id='text' name='text'></span></div>" End Function Public Function Close() m_ProgressBar.quit m_ProgressBar = Nothing End Function Private Function UpdateProgressBar() If m_PercentComplete = 0 Then m_StatusBarText = "" End If For n = m_CurrentStep to m_PercentComplete - 1 m_StatusBarText = m_StatusBarText & "|" m_ProgressBar.Document.GetElementById("statusbar").InnerHtml = m_StatusBarText m_ProgressBar.Document.title = n & "% Complete : " & m_Title m_ProgressBar.Document.GetElementById("pc").InnerHtml = n & "% Complete : " & m_Title wscript.sleep 10 Next m_ProgressBar.Document.GetElementById("statusbar").InnerHtml = m_StatusBarText m_ProgressBar.Document.title = m_PercentComplete & "% Complete : " & m_Title m_ProgressBar.Document.GetElementById("pc").InnerHtml = m_PercentComplete & "% Complete : " & m_Title m_ProgressBar.Document.GetElementById("text").InnerHtml = m_Text m_CurrentStep = m_PercentComplete End Function End Class
Example of Usage:
'Declare progressbar and percentage complete Dim pb Dim percentComplete 'Setup the initial progress bar Set pb = New ProgressBar percentComplete = 0 pb.SetTitle("Step 1 of 5") pb.SetText("Copying bin/Debug Folder") pb.Show() 'Loop to update the percent complete of the progress bar 'Just add the pb.Update in your code to update the bar 'Text can be updated as well by pb.SetText Do While percentComplete <= 100 wscript.sleep 500 pb.Update(percentComplete) percentComplete = percentComplete + 10 Loop wscript.sleep 3000 'This shows how you can use the code for multiple steps 'In a future iteration I will add a second bar to measure overall progress percentComplete = 0 pb.SetTitle("Step 2 of 5") pb.SetText("Copying bin/Release Folder") pb.Update(percentComplete) Do While percentComplete <= 100 wscript.sleep 500 pb.Update(percentComplete) percentComplete = percentComplete + 10 Loop pb.Close() wscript.quit
In a future release, I may add another progress bar to keep track of the steps that are completed. Feel free to use and modify this code as you desire.
Useful Links:
I was told today that using HTA with VBScript is a much more efficient way for a progress bar. I’ll be looking into it over the weekend, though at first glance it may require a second file.
Muy bueno, lo adapte y me funciono justo como queria, gracias por compartirlo.
i don’t speak english
This is simply awesome Jeff. Thank you for posting this code
I liked your idea very much and it was just what I was looking for. But after I slept on it, I came up with what I think is an improvement.
I replaced the text field with a table with one row and two fields. I set the left one with a bgcolor of blue and a width of 0. I set the right one with a bgcolor of white and a width of 300 px. I then changed the loop to find the id of the left and set the width to the step value times 3, and the width of the right to the 300 minus 3 times the step value. This way you get a pretty blue graphic bar filling from left to right. If using the depricated width and bgcolor is a hangup for you, I’m sure it could also be done with styles.
I impressed myself, at least.
Sounds like a good improvement Jeff C. I’ll have to think about it and post an updated version of this progress bar using your method or something similar.
Hello,
Thirst of all thanks for this progress-bar, it’s very handy to use
I have a small change proposal, so the update in progress(bar) is always rounded up to a one decimal integer. In this way the progress(bar) can’t breakdown when an multidecimal integer update value is used.
Change the line:
m_PercentComplete = percentComplete
To:
m_PercentComplete = Round(percentComplete, 0)
Cheers,
Peronnik Beijer
Excellent little application! Thanks for sharing this code.